
Using Switch Case statements in VBA Excel can significantly enhance the efficiency and readability of your code. Unlike some other programming languages, VBA does not have a built-in Switch Case statement like "Select Case" in some other languages, but it can be simulated using the Select Case
statement or by using If-ElseIf statements. However, for many scenarios, especially when dealing with a large number of cases, using the Choose
function or arrays can provide a similar functionality. Here's how you can use Switch Case in VBA Excel through various methods:
Simulating Switch Case Using Select Case
VBA does have a Select Case
statement that can serve the same purpose as a Switch Case statement. It's used to execute one block of code out of several alternatives.
Sub SampleSelectCase()
Dim MyVariable As Integer
MyVariable = 2
Select Case MyVariable
Case 1
MsgBox "You chose option 1"
Case 2
MsgBox "You chose option 2"
Case 3
MsgBox "You chose option 3"
Case Else
MsgBox "You chose an option that is not listed"
End Select
End Sub
Using If-ElseIf Statements as a Switch Case Alternative
If you prefer not to use Select Case
or if your conditions are more complex, you can use If-ElseIf statements to simulate a Switch Case.
Sub SampleIfElseIf()
Dim MyVariable As Integer
MyVariable = 2
If MyVariable = 1 Then
MsgBox "You chose option 1"
ElseIf MyVariable = 2 Then
MsgBox "You chose option 2"
ElseIf MyVariable = 3 Then
MsgBox "You chose option 3"
Else
MsgBox "You chose an option that is not listed"
End If
End Sub
Implementing Switch Case Using the Choose Function
The Choose
function is another way to simulate a Switch Case in VBA. It returns a value from a list of arguments based on the index number you provide.
Sub SampleChooseFunction()
Dim MyVariable As Integer
MyVariable = 2
Dim MyArray As Variant
MyArray = Array("You chose option 1", "You chose option 2", "You chose option 3")
MsgBox Choose(MyVariable, MyArray(0), MyArray(1), MyArray(2))
End Sub
Using Arrays as a Switch Case Alternative
You can also use arrays in a similar way to the Choose
function, especially if your conditions are not purely numeric.
Sub SampleArray()
Dim MyVariable As String
MyVariable = "option2"
Dim MyArray As Variant
MyArray = Array("option1", "You chose option 1", _
"option2", "You chose option 2", _
"option3", "You chose option 3")
For i = LBound(MyArray) To UBound(MyArray) Step 2
If MyArray(i) = MyVariable Then
MsgBox MyArray(i + 1)
Exit For
End If
Next i
End Sub
Image Representation of VBA Excel Switch Case
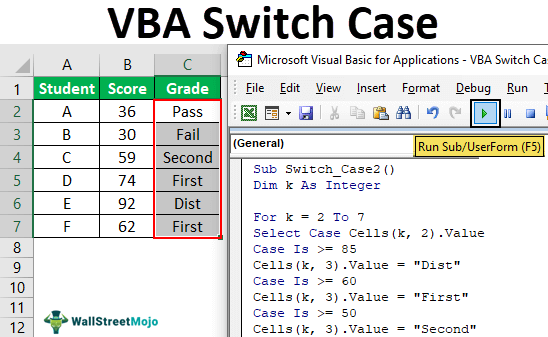
Each of these methods has its own advantages and can be chosen based on the specific needs of your VBA project in Excel. Whether you prefer the straightforward approach of Select Case
, the flexibility of If-ElseIf statements, or the concise nature of the Choose
function and arrays, there's a way to implement Switch Case logic that fits your programming style.
Gallery of VBA Switch Case Examples
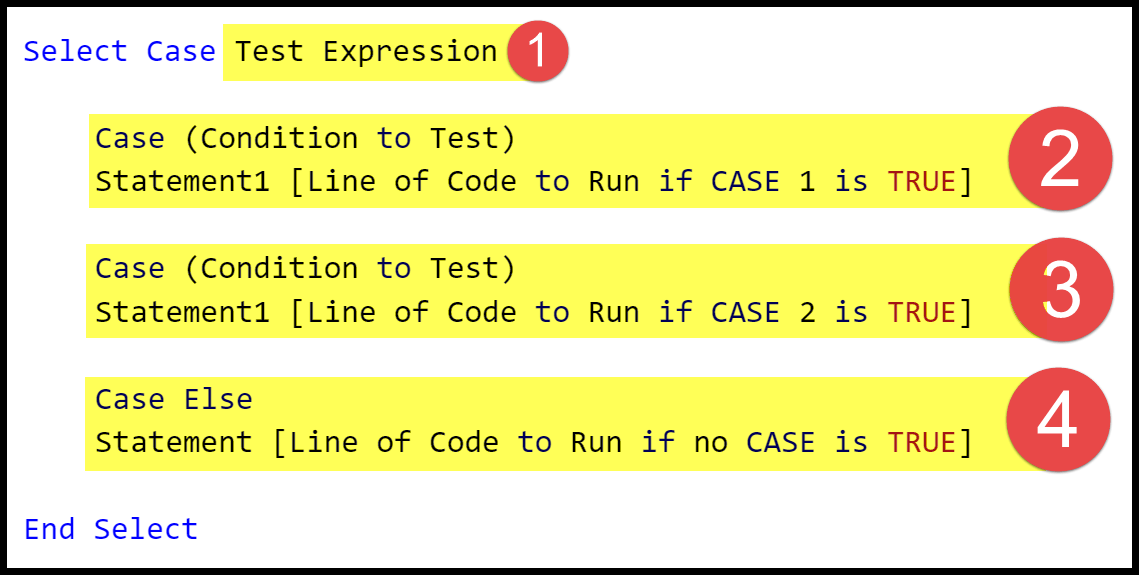

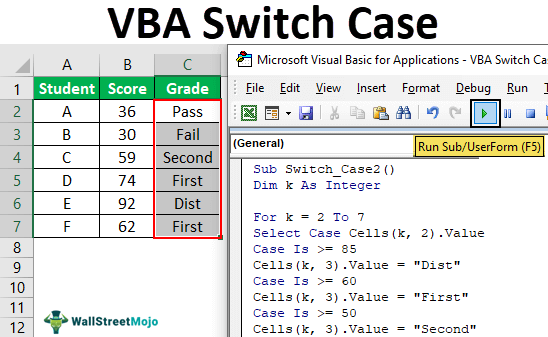
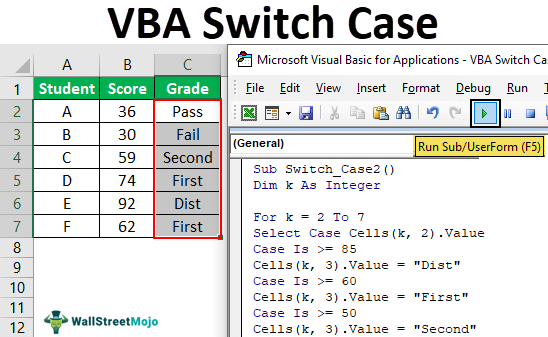
What is the primary use of Switch Case in VBA Excel?
+The primary use of Switch Case in VBA Excel is to execute one block of code out of several alternatives based on the value of an expression or variable.
How do you simulate a Switch Case in VBA Excel?
+You can simulate a Switch Case in VBA Excel using the Select Case statement, If-ElseIf statements, the Choose function, or arrays.
What is the advantage of using the Choose function?
+The Choose function provides a concise way to return a value from a list of arguments based on the index number you provide.