
The power of Excel VBA! Renaming sheets in Excel can be a tedious task, especially when dealing with multiple worksheets. Fortunately, Excel VBA provides several ways to rename sheets programmatically. In this article, we'll explore five ways to rename sheets in Excel VBA.
Why Rename Sheets in Excel VBA?
Renaming sheets in Excel VBA can be useful in various scenarios:
- Automating report generation: When generating reports, you may want to rename sheets to match the report's title or date.
- Organizing data: Renaming sheets can help organize data by categorizing worksheets based on their content.
- Creating templates: Renaming sheets can be part of a template creation process, where you want to rename sheets to match a specific template.
Method 1: Using the Worksheets
Collection
The Worksheets
collection is a straightforward way to rename sheets in Excel VBA. You can access a worksheet by its index or name and then use the Name
property to rename it.
Sub RenameSheetUsingWorksheetsCollection()
Worksheets(1).Name = "NewSheetName"
End Sub
In this example, we're renaming the first worksheet (index 1) to "NewSheetName".

Method 2: Using the ActiveSheet
Object
The ActiveSheet
object refers to the currently active worksheet. You can use this object to rename the active sheet.
Sub RenameActiveSheet()
ActiveSheet.Name = "NewSheetName"
End Sub
In this example, we're renaming the active sheet to "NewSheetName".
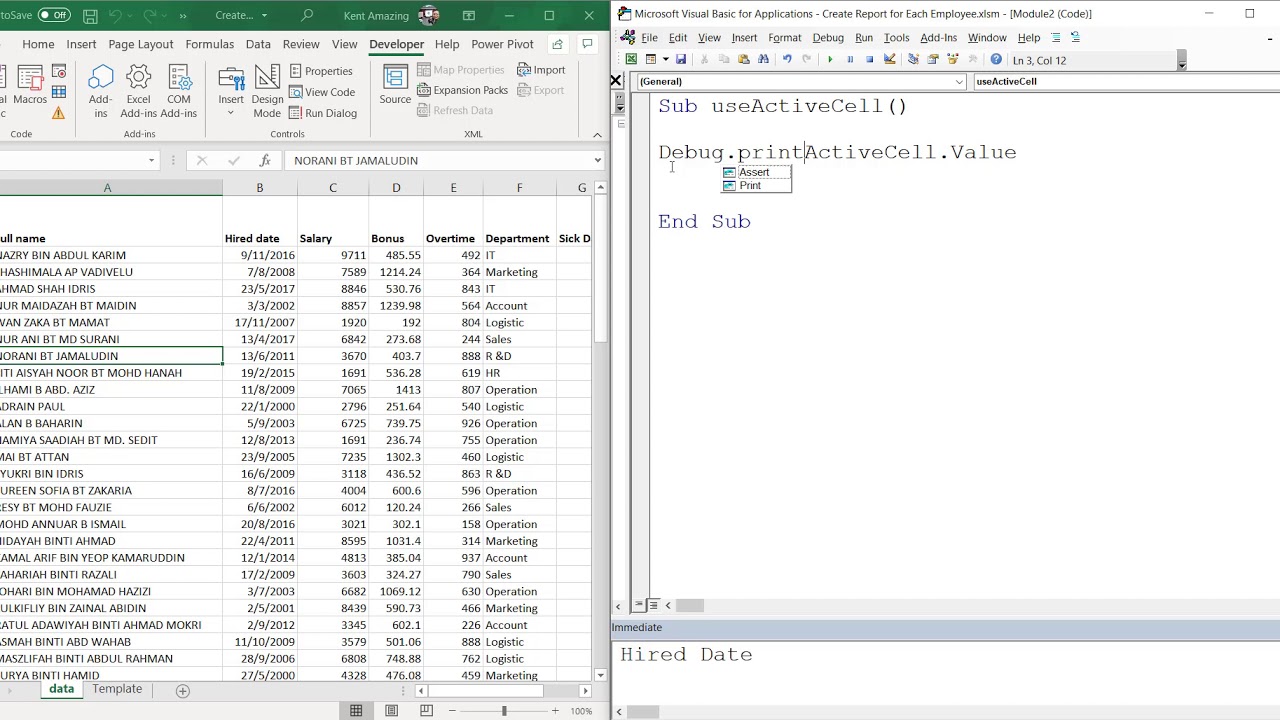
Method 3: Using the Worksheets
Collection with a Loop
If you want to rename multiple sheets, you can use a loop to iterate through the Worksheets
collection.
Sub RenameMultipleSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Name = "NewSheetName_" & ws.Index
Next ws
End Sub
In this example, we're renaming all worksheets in the active workbook by appending their index to "NewSheetName_".
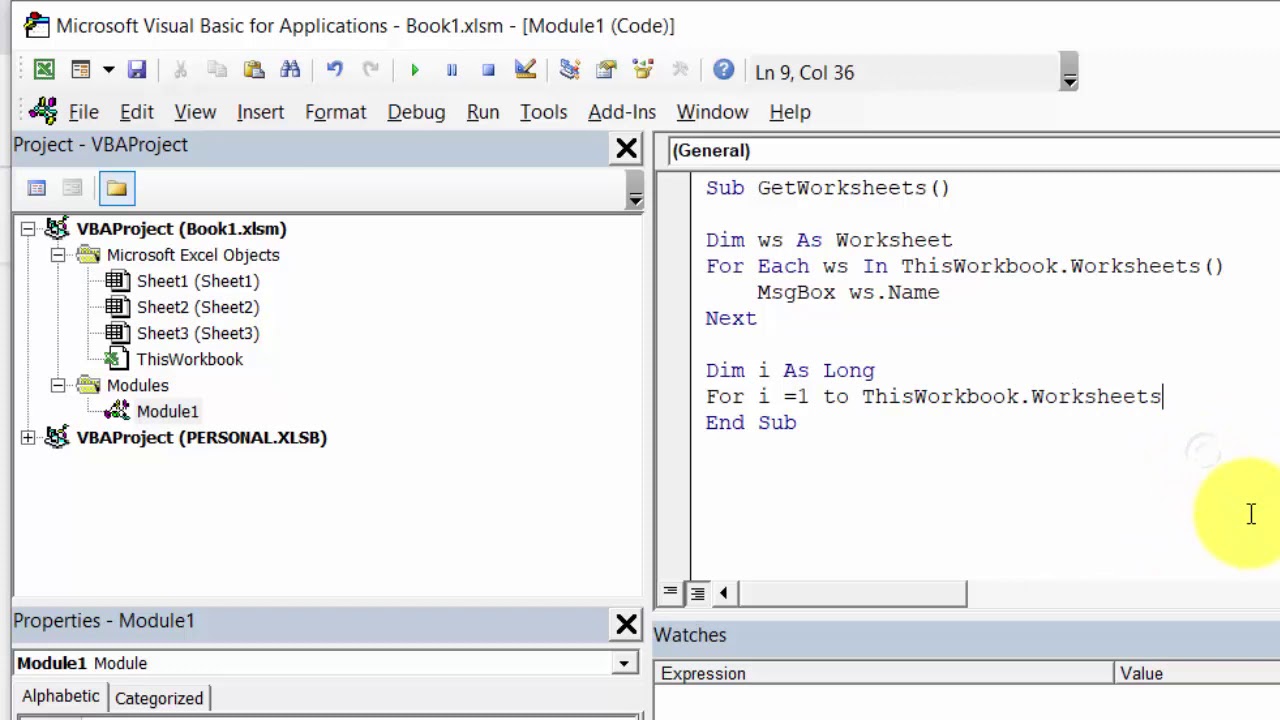
Method 4: Using the Worksheets
Collection with an Array
If you want to rename multiple sheets with specific names, you can use an array to store the new names.
Sub RenameMultipleSheetsUsingArray()
Dim newNames() As Variant
newNames = Array("NewSheetName1", "NewSheetName2", "NewSheetName3")
Dim i As Long
For i = LBound(newNames) To UBound(newNames)
Worksheets(i + 1).Name = newNames(i)
Next i
End Sub
In this example, we're renaming the first three worksheets with the names stored in the newNames
array.

Method 5: Using the Worksheets
Collection with a User-Defined Function
If you want to rename a sheet based on user input, you can create a user-defined function that takes the new name as an argument.
Function RenameSheet(newName As String) As Boolean
On Error Resume Next
Worksheets(1).Name = newName
RenameSheet = (Err.Number = 0)
Err.Clear
On Error GoTo 0
End Function
In this example, we're creating a function RenameSheet
that takes a new name as an argument and returns True
if the rename operation is successful.

Gallery of Excel VBA Rename Sheet Examples



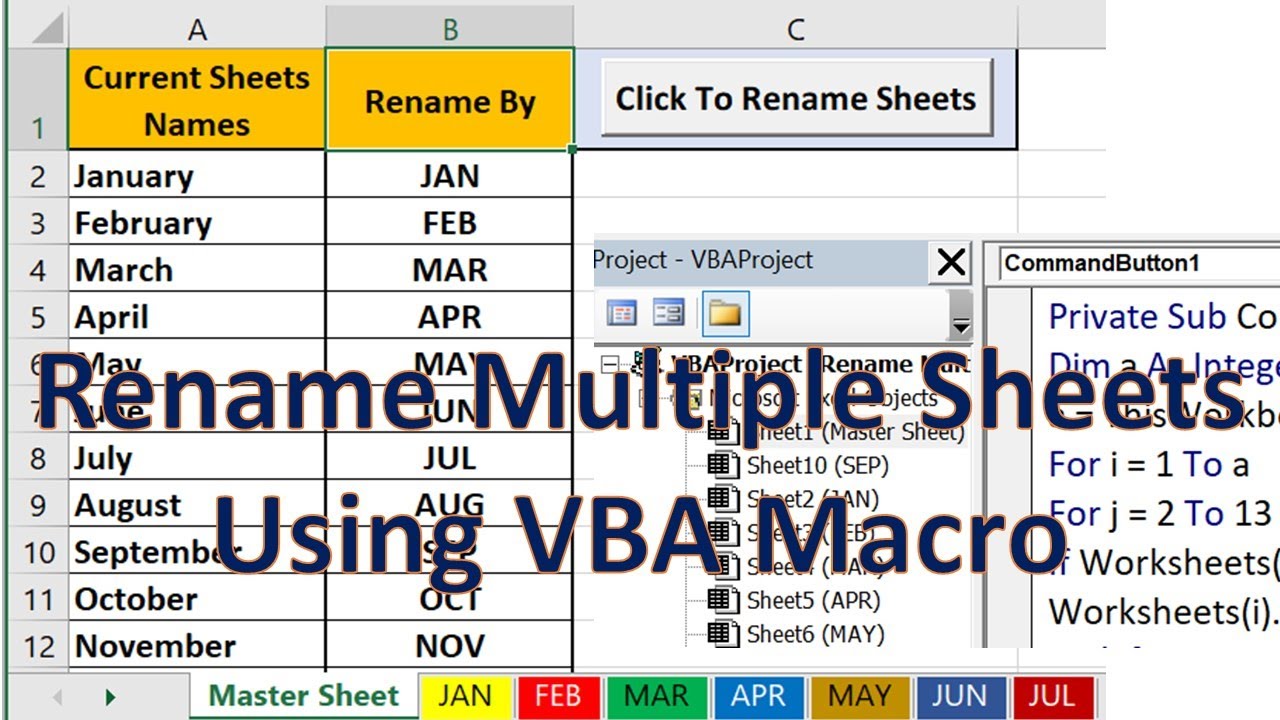
FAQs
How do I rename a sheet in Excel VBA?
+You can rename a sheet in Excel VBA using the `Worksheets` collection, `ActiveSheet` object, or a user-defined function.
Can I rename multiple sheets at once in Excel VBA?
+Yes, you can rename multiple sheets at once using a loop or an array.
How do I handle errors when renaming a sheet in Excel VBA?
+You can use error handling techniques such as `On Error Resume Next` and `Err.Clear` to handle errors when renaming a sheet.
We hope this article has provided you with a comprehensive guide on how to rename sheets in Excel VBA. Whether you're a beginner or an advanced user, these methods will help you automate your workflow and make your life easier.