
Excel VBA is a powerful tool that allows users to automate and customize their Excel spreadsheets. One of the most useful functions in Excel VBA is the Application.Match function, which allows users to search for a specific value in a range of cells and return the relative position of that value. In this article, we will explore seven ways to use the Application.Match function in Excel VBA.
The Application.Match function is a powerful tool that can be used in a variety of ways to improve the functionality of your Excel spreadsheets. By understanding how to use this function, you can automate tasks, improve efficiency, and reduce errors.
One of the most common uses of the Application.Match function is to search for a specific value in a range of cells. This can be useful when you need to find a specific piece of data in a large dataset.

1. Searching for a Specific Value
The Application.Match function can be used to search for a specific value in a range of cells. This can be useful when you need to find a specific piece of data in a large dataset. For example, if you have a list of employees and you want to find a specific employee's ID number, you can use the Application.Match function to search for that ID number in the list.
The syntax for the Application.Match function is as follows:
Application.Match(lookup_value, lookup_array, [match_type])
The lookup_value
is the value that you want to search for, the lookup_array
is the range of cells that you want to search in, and the [match_type]
is an optional parameter that specifies the type of match that you want to perform.
For example, if you want to search for the value "12345" in the range A1:A100, you can use the following code:
Application.Match("12345", Range("A1:A100"), 0)
This code will return the relative position of the value "12345" in the range A1:A100. If the value is not found, the function will return a #N/A error.
Using the Application.Match Function with Error Handling
When using the Application.Match function, it's a good idea to include error handling to deal with situations where the value is not found. You can use the IsError
function to check if the result of the Application.Match function is an error.
For example:
Sub SearchForValue()
Dim lookup_value As String
Dim lookup_array As Range
Dim result As Variant
lookup_value = "12345"
Set lookup_array = Range("A1:A100")
result = Application.Match(lookup_value, lookup_array, 0)
If IsError(result) Then
MsgBox "Value not found"
Else
MsgBox "Value found at position " & result
End If
End Sub
This code will search for the value "12345" in the range A1:A100 and display a message box indicating whether the value was found or not.
2. Using the Application.Match Function with Multiple Criteria
The Application.Match function can also be used to search for multiple criteria in a range of cells. This can be useful when you need to find a specific combination of values in a dataset.
To use the Application.Match function with multiple criteria, you can use the INDEX
and MATCH
functions together. The INDEX
function returns the value at a specified position in a range of cells, and the MATCH
function returns the relative position of a value in a range of cells.
For example, if you have a list of employees with their ID numbers, names, and departments, and you want to find the department of a specific employee, you can use the following code:
Sub FindDepartment()
Dim lookup_value As String
Dim lookup_array As Range
Dim department_array As Range
Dim result As Variant
lookup_value = "12345"
Set lookup_array = Range("A1:A100")
Set department_array = Range("C1:C100")
result = Application.Match(lookup_value, lookup_array, 0)
If IsError(result) Then
MsgBox "Value not found"
Else
MsgBox "Department: " & Application.Index(department_array, result)
End If
End Sub
This code will search for the value "12345" in the range A1:A100 and return the department of the employee with that ID number.

3. Using the Application.Match Function with Wildcards
The Application.Match function can also be used to search for values with wildcards. Wildcards are special characters that can be used to represent unknown or variable characters in a search string.
To use the Application.Match function with wildcards, you can use the LIKE
operator in your search string. The LIKE
operator allows you to search for values that match a specified pattern.
For example, if you want to search for all employees with names that start with the letter "J", you can use the following code:
Sub SearchForNames()
Dim lookup_value As String
Dim lookup_array As Range
Dim result As Variant
lookup_value = "J*"
Set lookup_array = Range("B1:B100")
result = Application.Match(lookup_value, lookup_array, 0)
If IsError(result) Then
MsgBox "Value not found"
Else
MsgBox "Value found at position " & result
End If
End Sub
This code will search for all values in the range B1:B100 that start with the letter "J" and return the relative position of the first match.
Using the Application.Match Function with Regular Expressions
The Application.Match function can also be used to search for values with regular expressions. Regular expressions are a powerful way to search for patterns in strings.
To use the Application.Match function with regular expressions, you can use the RegExp
object in your VBA code. The RegExp
object allows you to create and use regular expressions in your VBA code.
For example, if you want to search for all employees with names that contain the letter "J" or "K", you can use the following code:
Sub SearchForNames()
Dim lookup_value As String
Dim lookup_array As Range
Dim result As Variant
Dim regex As Object
Set regex = CreateObject("VBScript.RegExp")
regex.Pattern = "[JK]"
regex.IgnoreCase = True
lookup_value = "J*K"
Set lookup_array = Range("B1:B100")
result = Application.Match(lookup_value, lookup_array, 0)
If IsError(result) Then
MsgBox "Value not found"
Else
MsgBox "Value found at position " & result
End If
End Sub
This code will search for all values in the range B1:B100 that contain the letter "J" or "K" and return the relative position of the first match.
4. Using the Application.Match Function with Dates
The Application.Match function can also be used to search for dates in a range of cells. This can be useful when you need to find a specific date in a dataset.
To use the Application.Match function with dates, you can use the Date
data type in your VBA code. The Date
data type allows you to work with dates in your VBA code.
For example, if you want to search for all employees with birthdays on a specific date, you can use the following code:
Sub SearchForBirthdays()
Dim lookup_value As Date
Dim lookup_array As Range
Dim result As Variant
lookup_value = #1/1/1990#
Set lookup_array = Range("C1:C100")
result = Application.Match(lookup_value, lookup_array, 0)
If IsError(result) Then
MsgBox "Value not found"
Else
MsgBox "Value found at position " & result
End If
End Sub
This code will search for all values in the range C1:C100 that match the date January 1, 1990, and return the relative position of the first match.
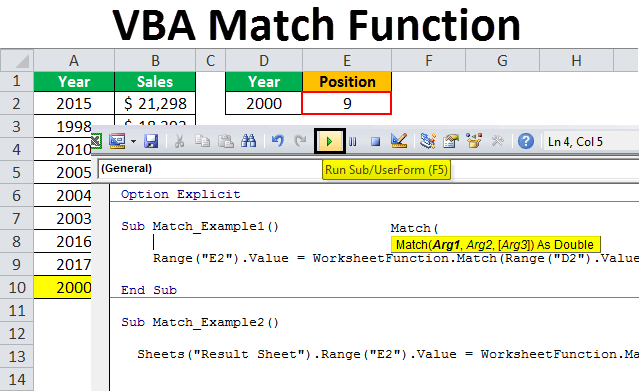
5. Using the Application.Match Function with Times
The Application.Match function can also be used to search for times in a range of cells. This can be useful when you need to find a specific time in a dataset.
To use the Application.Match function with times, you can use the Time
data type in your VBA code. The Time
data type allows you to work with times in your VBA code.
For example, if you want to search for all employees with start times on a specific time, you can use the following code:
Sub SearchForStartTimes()
Dim lookup_value As Date
Dim lookup_array As Range
Dim result As Variant
lookup_value = #8:00:00 AM#
Set lookup_array = Range("D1:D100")
result = Application.Match(lookup_value, lookup_array, 0)
If IsError(result) Then
MsgBox "Value not found"
Else
MsgBox "Value found at position " & result
End If
End Sub
This code will search for all values in the range D1:D100 that match the time 8:00:00 AM and return the relative position of the first match.
6. Using the Application.Match Function with Arrays
The Application.Match function can also be used to search for values in arrays. This can be useful when you need to find a specific value in an array.
To use the Application.Match function with arrays, you can use the Array
data type in your VBA code. The Array
data type allows you to work with arrays in your VBA code.
For example, if you want to search for a specific value in an array, you can use the following code:
Sub SearchForValueInArray()
Dim lookup_value As Variant
Dim lookup_array As Variant
Dim result As Variant
lookup_value = "12345"
lookup_array = Array("12345", "67890", "11111")
result = Application.Match(lookup_value, lookup_array, 0)
If IsError(result) Then
MsgBox "Value not found"
Else
MsgBox "Value found at position " & result
End If
End Sub
This code will search for the value "12345" in the array and return the relative position of the value.

7. Using the Application.Match Function with Worksheets
The Application.Match function can also be used to search for values in worksheets. This can be useful when you need to find a specific value in a worksheet.
To use the Application.Match function with worksheets, you can use the Worksheet
data type in your VBA code. The Worksheet
data type allows you to work with worksheets in your VBA code.
For example, if you want to search for a specific value in a worksheet, you can use the following code:
Sub SearchForValueInWorksheet()
Dim lookup_value As Variant
Dim lookup_array As Range
Dim result As Variant
lookup_value = "12345"
Set lookup_array = ThisWorkbook.Worksheets("Sheet1").Range("A1:A100")
result = Application.Match(lookup_value, lookup_array, 0)
If IsError(result) Then
MsgBox "Value not found"
Else
MsgBox "Value found at position " & result
End If
End Sub
This code will search for the value "12345" in the range A1:A100 in the worksheet "Sheet1" and return the relative position of the value.




What is the Application.Match function in Excel VBA?
+The Application.Match function is a powerful tool in Excel VBA that allows users to search for a specific value in a range of cells and return the relative position of that value.
How do I use the Application.Match function with multiple criteria?
+To use the Application.Match function with multiple criteria, you can use the INDEX and MATCH functions together. The INDEX function returns the value at a specified position in a range of cells, and the MATCH function returns the relative position of a value in a range of cells.
Can I use the Application.Match function with wildcards?
+Yes, you can use the Application.Match function with wildcards. To do this, you can use the LIKE operator in your search string. The LIKE operator allows you to search for values that match a specified pattern.
We hope this article has provided you with a comprehensive guide to using the Application.Match function in Excel VBA. Whether you're a beginner or an advanced user, this function can be a powerful tool in your VBA arsenal. Remember to always use error handling and to test your code thoroughly to ensure that it works as expected. Happy coding!