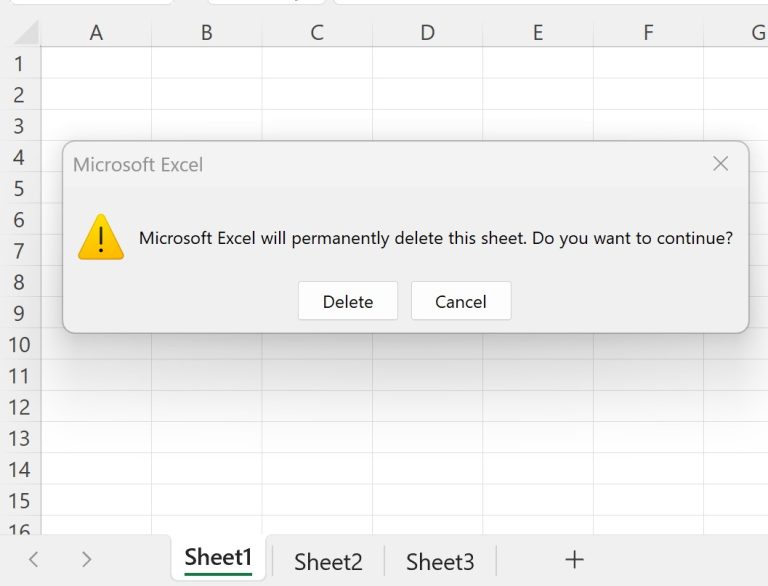
Deleting sheets in Excel VBA can be a straightforward process, but the warning messages that pop up can be annoying. These warnings are designed to prevent accidental deletion of important data. However, when working with VBA, you often want to automate tasks without interruptions. Here are three ways to delete sheets in VBA without warning messages.
Method 1: Using the `Application.DisplayAlerts` Property
One way to suppress warning messages when deleting sheets is by setting the Application.DisplayAlerts
property to False
. This property determines whether Excel displays alerts and warning messages. By setting it to False
, you can prevent the warning message from appearing when deleting a sheet.
Sub DeleteSheetWithoutWarning1()
Application.DisplayAlerts = False
Sheets("Sheet1").Delete
Application.DisplayAlerts = True
End Sub
In this example, Application.DisplayAlerts
is set to False
before deleting the sheet, and then set back to True
afterwards. This ensures that the warning message is only suppressed for the duration of the deletion process.

Method 2: Using the `Application.Sheets.Delete` Method with the `Alert` Parameter
Another way to delete sheets without warning messages is by using the Application.Sheets.Delete
method with the Alert
parameter set to xlNoAlert
. This method allows you to delete a sheet without prompting the user.
Sub DeleteSheetWithoutWarning2()
Application.Sheets.Delete "Sheet1", xlNoAlert
End Sub
In this example, the Application.Sheets.Delete
method is used to delete the sheet named "Sheet1", with the Alert
parameter set to xlNoAlert
. This suppresses the warning message and deletes the sheet without prompting the user.

Method 3: Using Error Handling
The third method involves using error handling to suppress the warning message. This method is useful when you're not sure if the sheet exists or not.
Sub DeleteSheetWithoutWarning3()
On Error Resume Next
Sheets("Sheet1").Delete
On Error GoTo 0
End Sub
In this example, the On Error Resume Next
statement is used to suppress any error messages that may occur when deleting the sheet. If the sheet does not exist, the code will simply move on to the next line without prompting the user.

Conclusion
Deleting sheets in Excel VBA can be done in several ways, each with its own advantages and disadvantages. By using one of the methods outlined above, you can suppress the warning messages that normally appear when deleting sheets. Whether you choose to use the Application.DisplayAlerts
property, the Application.Sheets.Delete
method with the Alert
parameter, or error handling, the end result is the same: a seamless and automated deletion process.




What is the difference between the three methods?
+The three methods differ in how they suppress the warning message. The first method uses the `Application.DisplayAlerts` property, the second method uses the `Application.Sheets.Delete` method with the `Alert` parameter, and the third method uses error handling. Each method has its own advantages and disadvantages.
Which method is the most efficient?
+The most efficient method depends on the specific use case. If you need to delete multiple sheets, the first method may be the most efficient. If you need to delete a single sheet, the second method may be the most efficient. If you're not sure if the sheet exists or not, the third method may be the most efficient.
What are the best practices for deleting sheets in VBA?
+Best practices for deleting sheets in VBA include using one of the methods outlined above, being mindful of the `Application.DisplayAlerts` property, and using error handling to handle unexpected errors.