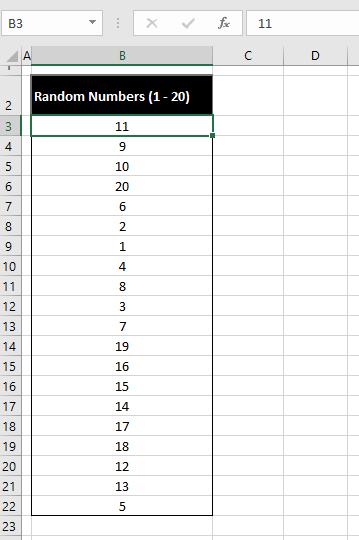
In various Excel VBA applications, generating random numbers can be a necessity for simulations, modeling, or even for creating test data. Excel VBA offers several methods to generate random numbers, each with its own advantages and specific use cases. Here are five common ways to generate random numbers in Excel VBA, along with examples of how to use them in your projects.
1. Using the Rnd() Function
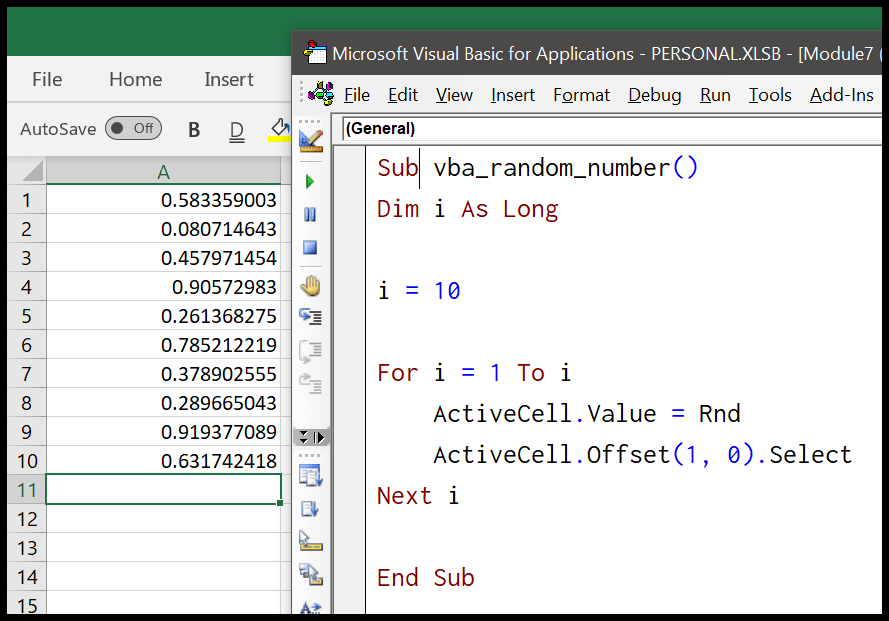
The Rnd()
function is the most straightforward way to generate random numbers in Excel VBA. It returns a random number between 0 and 1. You can scale this value to get a random number within a specific range. However, Rnd()
uses the system clock as its seed value, so you might notice patterns in the sequence of random numbers generated consecutively without any significant delay. This issue can be addressed by explicitly setting a seed value using the Randomize
statement.
Here's an example of using Rnd()
to generate a random number between 1 and 100:
Sub GenerateRandomNumber()
Randomize 'Setting a random seed based on the current time
Dim randomNumber As Double
randomNumber = Int((100 * Rnd) + 1) 'Generate a random integer between 1 and 100
MsgBox randomNumber
End Sub
2. Using the WorksheetFunction.RandBetween Method
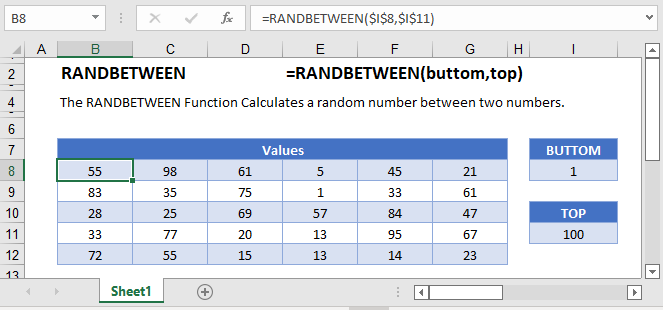
For generating random integers within a specified range, the RandBetween
method from the WorksheetFunction
object is a handy option. It's a bit more straightforward than manually scaling the result of Rnd()
, as it directly returns a random integer within the specified bounds.
Here's how you can use RandBetween
to generate a random integer between 1 and 100:
Sub GenerateRandomInteger()
Dim randomNumber As Integer
randomNumber = WorksheetFunction.RandBetween(1, 100) 'Generate a random integer between 1 and 100
MsgBox randomNumber
End Sub
3. Utilizing the Cryptographic API for Truly Random Numbers
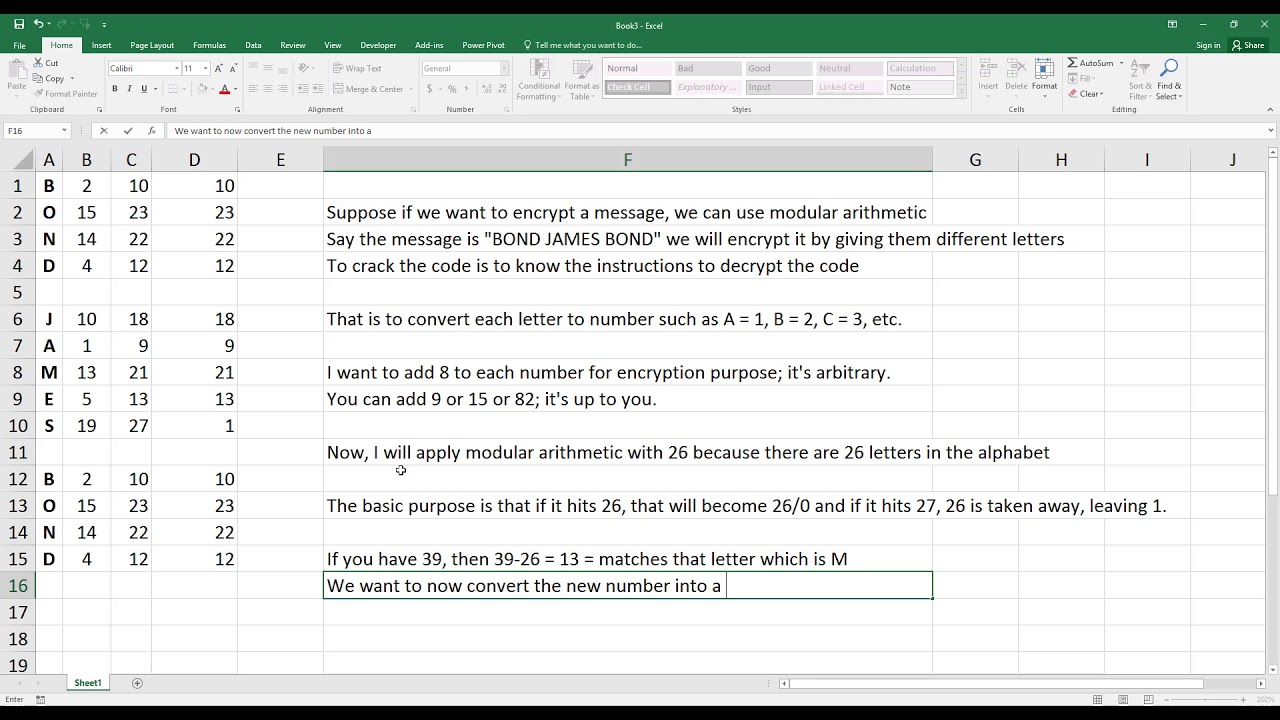
When the highest level of randomness is required (for instance, in simulations or cryptographic applications), relying on the Windows Cryptographic API can provide truly random numbers. This involves using the advapi32.dll
library and its functions, which can be a bit more complex to set up and use.
Here's a simplified example of using the Cryptographic API to generate a truly random integer:
Private Declare PtrSafe Function CryptAcquireContext Lib "advapi32.dll" _
Alias "CryptAcquireContextA" (ByRef phProv As Long, _
ByVal container As String, _
ByVal provider As String, _
ByVal provType As Long, _
ByVal flags As Long) As Long
Private Declare PtrSafe Function CryptGenRandom Lib "advapi32.dll" _
(ByVal hProv As Long, _
ByVal dwLen As Long, _
ByVal pbBuffer As Long) As Long
Private Declare PtrSafe Function CryptReleaseContext Lib "advapi32.dll" _
(ByVal hProv As Long, _
ByVal flags As Long) As Long
Sub GenerateTrulyRandomInteger()
Const PROV_RSA_FULL = 1
Const CRYPT_VERIFYCONTEXT = &HF0000000
Const CRYPT_DELETEKEYSET = &H10
Dim hCryptProv As Long
Dim randomBuffer() As Byte
Dim randomValue As Long
'Acquire a handle to a key container
If CryptAcquireContext(hCryptProv, 0&, 0&, PROV_RSA_FULL, CRYPT_VERIFYCONTEXT) <> 0 Then
'Generate random bytes
ReDim randomBuffer(3)
If CryptGenRandom(hCryptProv, 4, VarPtr(randomBuffer(0))) <> 0 Then
'Convert the first four bytes into an integer
randomValue = CLng(randomBuffer(0)) + (CLng(randomBuffer(1)) * 256) + (CLng(randomBuffer(2)) * 65536)
'Ensure the value is within the desired range (0 to 100)
randomValue = randomValue Mod 101
MsgBox randomValue
End If
'Release the provider handle
If CryptReleaseContext(hCryptProv, 0) = 0 Then MsgBox "Failed to release context"
Else
MsgBox "Failed to acquire context"
End If
End Sub
4. Leveraging VBA's Random Number Generation from External Sources
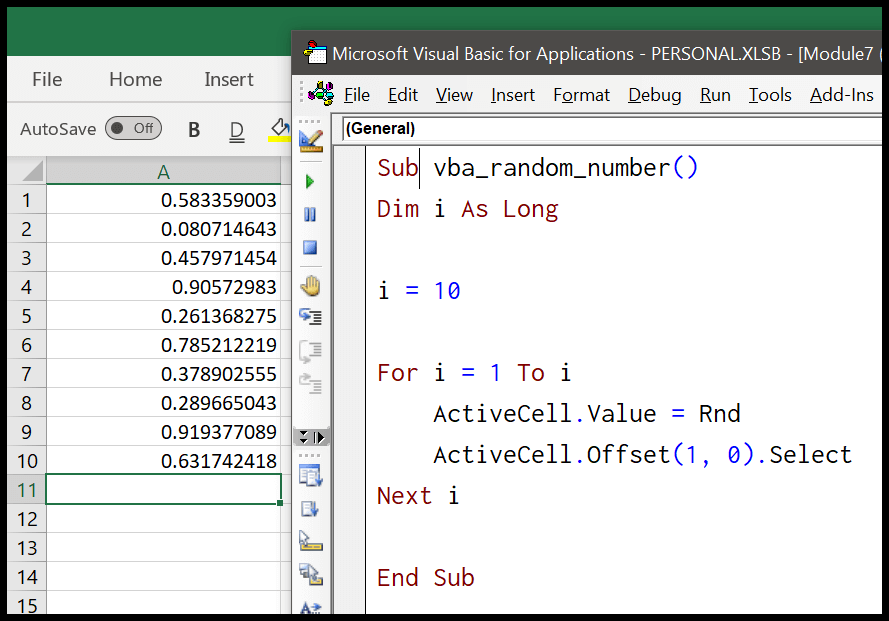
There are instances where you might want to utilize external resources or web services that generate random numbers. This could be due to the nature of your application or the specific requirements for randomness. In such cases, you can use VBA to query these external sources and obtain the random numbers.
Here's a basic example of how you might fetch a random number from an external API:
Sub GenerateRandomNumberFromAPI()
Dim xmlHttp As Object
Set xmlHttp = CreateObject("MSXML2.XMLHTTP")
xmlHttp.Open "GET", "https://www.random.org/integers/?num=1&min=1&max=100&col=1&base=10&format=plain&rnd=new", False
xmlHttp.Send
Dim randomNumber As String
randomNumber = xmlHttp.responseText
MsgBox randomNumber
End Sub
Please note that the external API's terms of service and the specifics of how to use it (e.g., formatting the query) will dictate the precise code needed.
5. Using VBA's own Random Number Generator Function with a Static Seed for Reproducibility
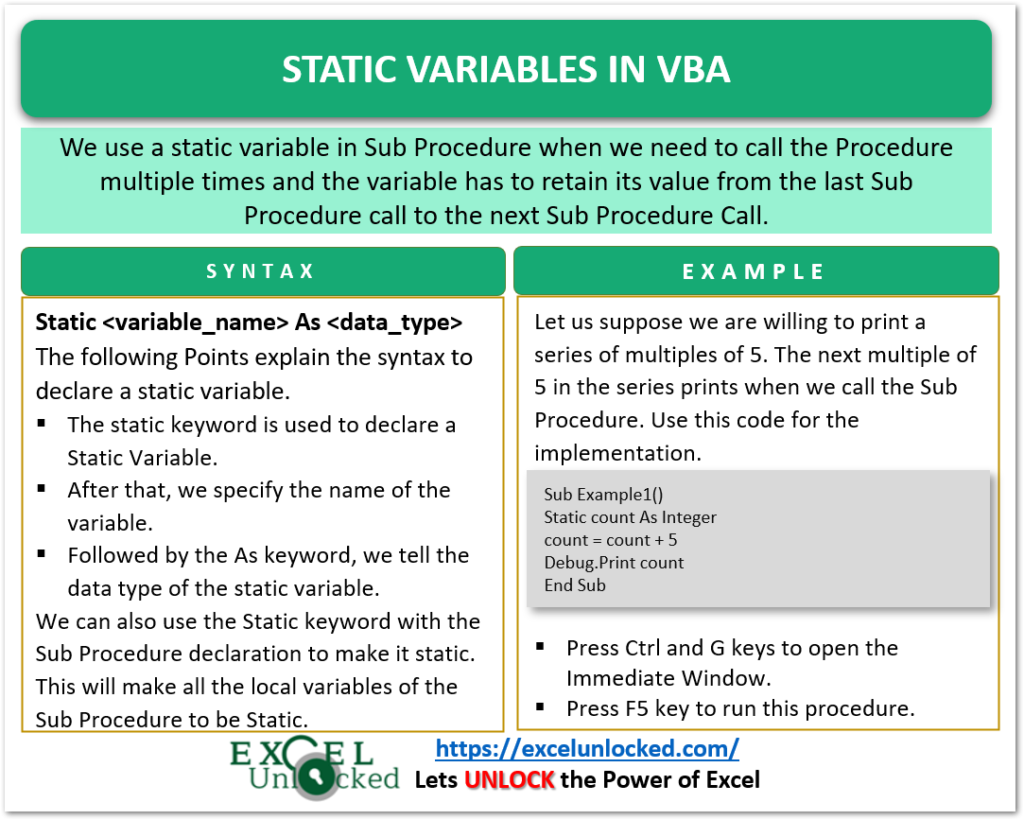
When reproducibility is key (for example, in statistical analysis or simulation studies where you want to reproduce the exact sequence of random numbers), using a static seed for the random number generator is crucial. VBA allows you to set the seed using the Randomize
statement with a specific seed value.
Here's how to generate random numbers with a static seed:
Sub GenerateReproducibleRandomNumbers()
Randomize 12345 'Setting a static seed
Dim randomNumber As Double
randomNumber = Rnd() 'Generate a random double
MsgBox randomNumber
End Sub
By using these different methods, you can generate random numbers in Excel VBA tailored to your specific application needs.
Wrapping Up
Generating random numbers in Excel VBA can be a straightforward task, but the approach can vary depending on your specific requirements. From the simplicity of Rnd()
and RandBetween
to the complexity of utilizing external APIs or the Cryptographic API for high entropy, each method has its place. Whether you're developing a game, a simulation, or simply need test data, understanding these methods will help you make informed decisions about how best to incorporate randomness into your VBA projects.
Gallery of Random Number Generation
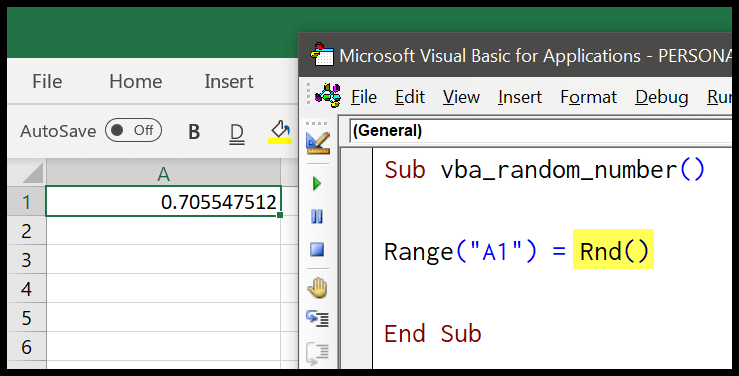
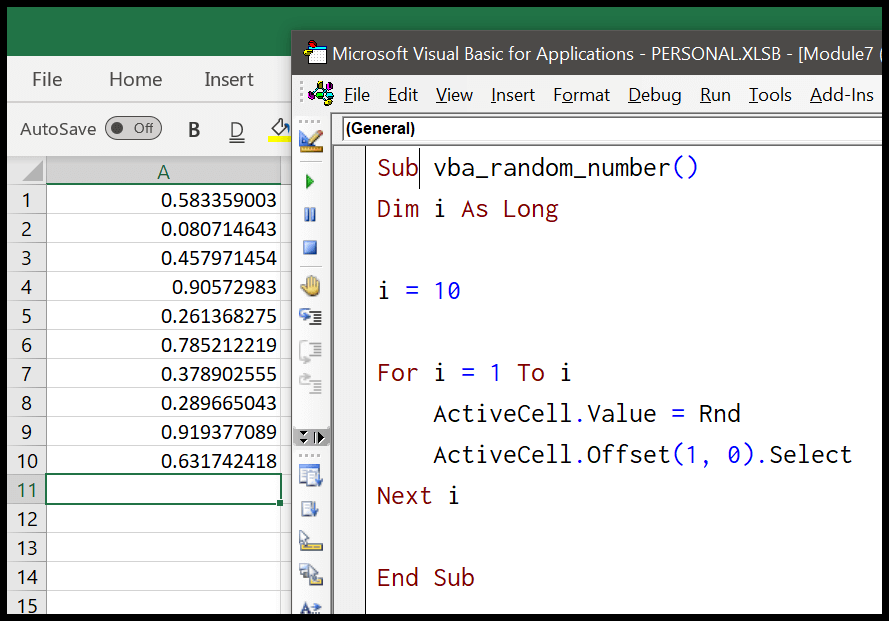
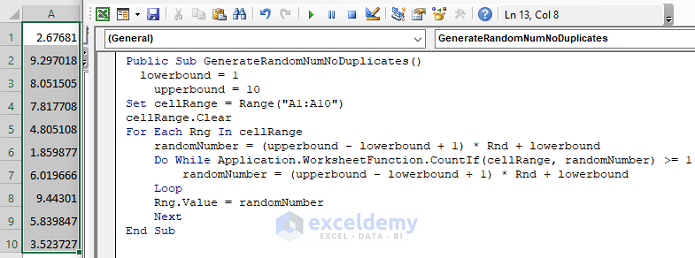
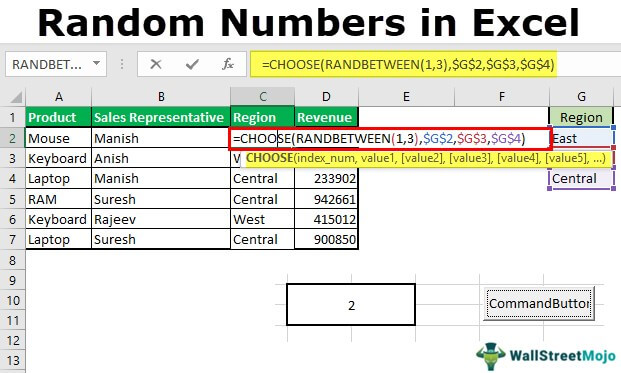
FAQs
How do I generate random numbers in Excel VBA?
+You can generate random numbers in Excel VBA using the `Rnd()` function, `RandBetween` method, or by leveraging external sources like the Cryptographic API.
What is the difference between `Rnd()` and `RandBetween` in VBA?
+`Rnd()` generates a random number between 0 and 1, while `RandBetween` generates a random integer within a specified range.
How do I make my random number generation reproducible in VBA?
+You can set a static seed using the `Randomize` statement with a specific seed value to ensure reproducibility.