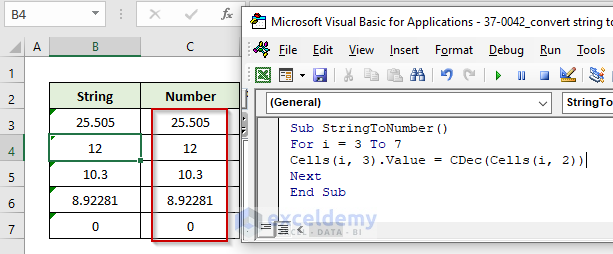
Converting strings to numbers in VBA can be a frustrating task, especially when dealing with data from external sources. However, with the right techniques and tools, it can be made easy. In this article, we will explore the different methods of converting strings to numbers in VBA, including using built-in functions, formatting, and error handling.
Understanding the Problem
In VBA, strings and numbers are two different data types. While strings are represented as text, numbers are represented as numerical values. When you try to perform arithmetic operations on a string, VBA will throw an error. Therefore, it is essential to convert strings to numbers before performing any mathematical operations.
Method 1: Using the Val Function
The Val function is a built-in VBA function that converts a string to a number. It is the most straightforward method of converting strings to numbers.
Dim myString As String
myString = "123"
Dim myNumber As Double
myNumber = Val(myString)
The Val function works by reading the string from left to right and converting the characters to numbers. It ignores any non-numeric characters, except for a decimal point or a negative sign at the beginning.
Method 2: Using the CDbl Function
The CDbl function is another built-in VBA function that converts a string to a double-precision number.
Dim myString As String
myString = "123.45"
Dim myNumber As Double
myNumber = CDbl(myString)
The CDbl function is more robust than the Val function, as it can handle decimal points and negative numbers.
Method 3: Using the Format Function
The Format function is a built-in VBA function that formats a string according to a specified format. It can also be used to convert strings to numbers.
Dim myString As String
myString = "123"
Dim myNumber As Double
myNumber = Format(myString, "Standard")
The Format function works by applying a standard number format to the string. This method is useful when you need to perform additional formatting on the number.
Method 4: Using Error Handling
Error handling is an essential part of converting strings to numbers in VBA. When working with external data, it is common to encounter strings that cannot be converted to numbers. In such cases, VBA will throw an error. To handle these errors, you can use the On Error statement.
On Error Resume Next
Dim myString As String
myString = "abc"
Dim myNumber As Double
myNumber = Val(myString)
If Err.Number <> 0 Then
MsgBox "Error converting string to number", vbExclamation
End If
On Error GoTo 0
The On Error statement allows you to catch and handle errors in your code. By using the Err.Number property, you can check if an error occurred and take corrective action.
Method 5: Using Regular Expressions
Regular expressions are a powerful tool for manipulating strings in VBA. They can be used to extract numbers from strings and convert them to numbers.
Dim myString As String
myString = "abc123def"
Dim myNumber As Double
myNumber = RegExExtract(myString, "\d+")
The RegExExtract function uses regular expressions to extract numbers from the string. This method is useful when you need to extract numbers from complex strings.
Conclusion
Converting strings to numbers in VBA can be made easy by using the right techniques and tools. By understanding the different methods available, you can choose the best approach for your specific needs. Whether you are working with external data or manipulating strings in your code, these methods will help you to convert strings to numbers with ease.
Gallery of VBA String to Number Conversion

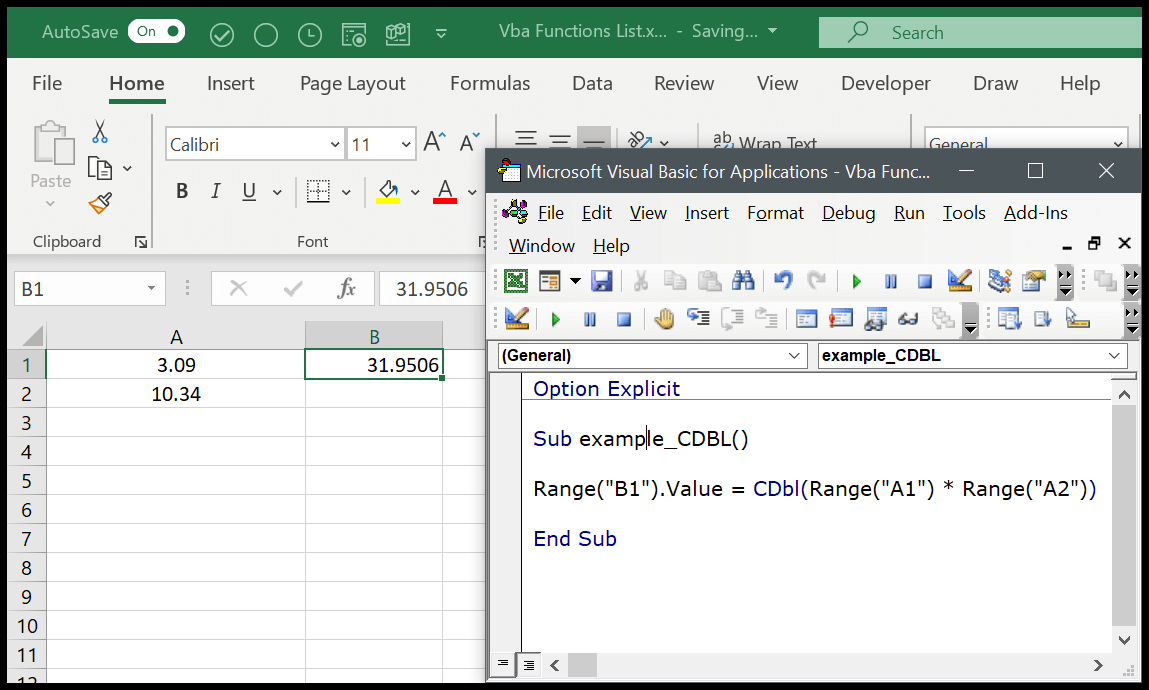


FAQs
What is the difference between the Val and CDbl functions in VBA?
+The Val function converts a string to a variant, while the CDbl function converts a string to a double-precision number. The CDbl function is more robust and can handle decimal points and negative numbers.
How do I handle errors when converting strings to numbers in VBA?
+Use the On Error statement to catch and handle errors. You can use the Err.Number property to check if an error occurred and take corrective action.
Can I use regular expressions to extract numbers from strings in VBA?
+Yes, you can use regular expressions to extract numbers from strings in VBA. The RegExExtract function uses regular expressions to extract numbers from the string.