
Opening a text file in VBA (Visual Basic for Applications) is a fundamental task that can be challenging for beginners. However, with the right guidance, you can easily learn how to read and write text files using VBA. In this article, we will explore the different methods of opening a text file in VBA, including the File System Object (FSO) and the Open statement.
Why Open a Text File in VBA?
There are many reasons why you might need to open a text file in VBA. Some common scenarios include:
- Reading data from a text file to populate a spreadsheet or database
- Writing data from a spreadsheet or database to a text file
- Parsing and processing text data
- Creating log files or error reports
Method 1: Using the File System Object (FSO)
The File System Object (FSO) is a powerful tool in VBA that allows you to interact with the file system. You can use the FSO to open, read, and write text files. Here's an example of how to use the FSO to open a text file:

Sub OpenTextFileUsingFSO()
Dim fso As Object
Dim txtFile As Object
' Create a new instance of the File System Object
Set fso = CreateObject("Scripting.FileSystemObject")
' Open the text file
Set txtFile = fso.OpenTextFile("C:\Example\Example.txt", 1)
' Read the contents of the text file
Dim contents As String
contents = txtFile.ReadAll
' Close the text file
txtFile.Close
' Release the objects
Set txtFile = Nothing
Set fso = Nothing
End Sub
Method 2: Using the Open Statement
The Open statement is another way to open a text file in VBA. This method is more straightforward than the FSO method, but it has some limitations. Here's an example of how to use the Open statement to open a text file:
Sub OpenTextFileUsingOpenStatement()
Dim contents As String
' Open the text file
Open "C:\Example\Example.txt" For Input As #1
' Read the contents of the text file
contents = Input$(LOF(1), #1)
' Close the text file
Close #1
End Sub
Reading and Writing Text Files
Once you have opened a text file, you can read and write data to it. Here are some examples of how to read and write text files using VBA:
Reading a Text File
To read a text file, you can use the ReadAll
method of the TextStream
object (FSO method) or the Input
function (Open statement method). Here's an example of how to read a text file using the FSO method:
Sub ReadTextFile()
Dim fso As Object
Dim txtFile As Object
Dim contents As String
' Create a new instance of the File System Object
Set fso = CreateObject("Scripting.FileSystemObject")
' Open the text file
Set txtFile = fso.OpenTextFile("C:\Example\Example.txt", 1)
' Read the contents of the text file
contents = txtFile.ReadAll
' Close the text file
txtFile.Close
' Release the objects
Set txtFile = Nothing
Set fso = Nothing
End Sub
Writing a Text File
To write a text file, you can use the Write
method of the TextStream
object (FSO method) or the Print
statement (Open statement method). Here's an example of how to write a text file using the FSO method:
Sub WriteTextFile()
Dim fso As Object
Dim txtFile As Object
' Create a new instance of the File System Object
Set fso = CreateObject("Scripting.FileSystemObject")
' Open the text file
Set txtFile = fso.OpenTextFile("C:\Example\Example.txt", 2)
' Write to the text file
txtFile.Write "Hello, World!"
' Close the text file
txtFile.Close
' Release the objects
Set txtFile = Nothing
Set fso = Nothing
End Sub

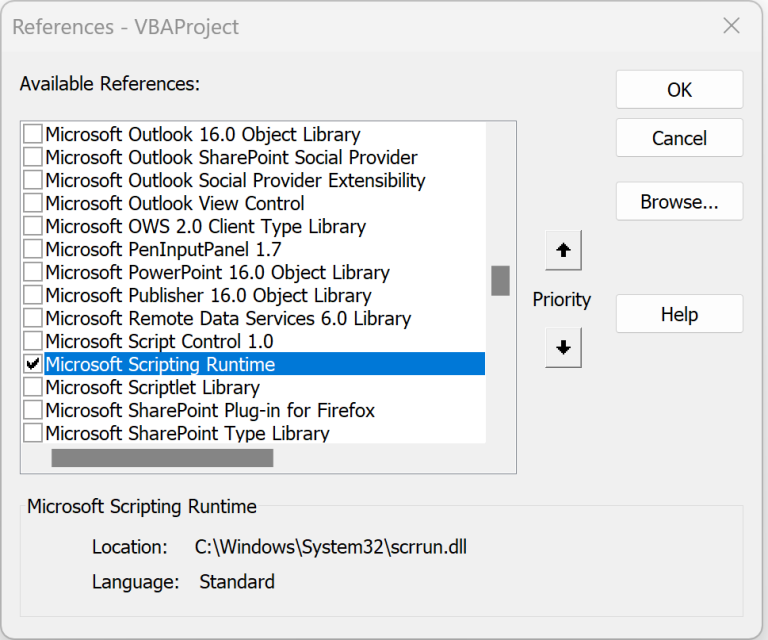


What is the File System Object (FSO) in VBA?
+The File System Object (FSO) is a powerful tool in VBA that allows you to interact with the file system. You can use the FSO to open, read, and write text files.
How do I open a text file in VBA using the Open statement?
+To open a text file using the Open statement, use the syntax `Open "filename" For Input As #1`. Replace "filename" with the path and name of the text file you want to open.
How do I read a text file in VBA using the FSO method?
+To read a text file using the FSO method, use the `ReadAll` method of the `TextStream` object. For example: `contents = txtFile.ReadAll`.