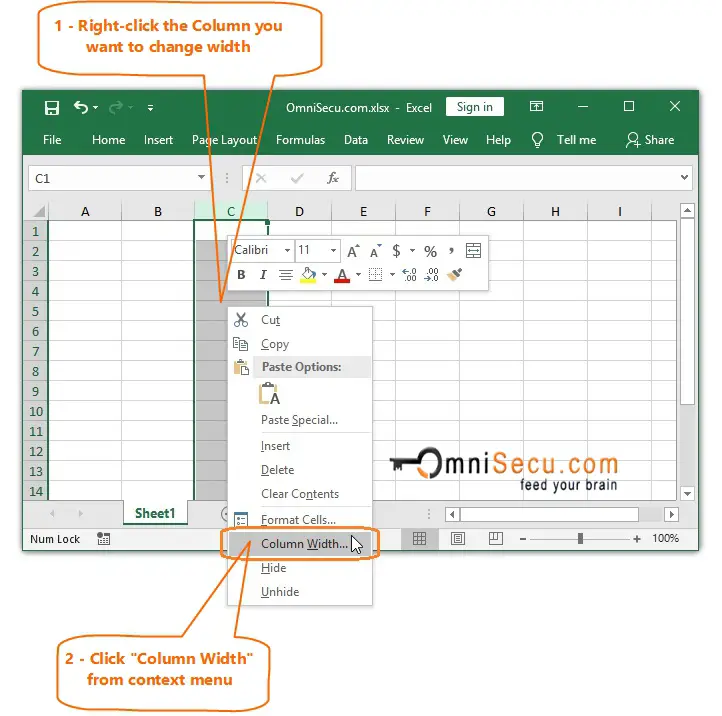
The world of Excel VBA is full of endless possibilities. One of the most commonly used tasks in Excel VBA is adjusting column widths. Whether you're creating a new spreadsheet or editing an existing one, changing column widths can greatly impact the overall appearance and functionality of your worksheet. In this article, we will explore five different ways to change column widths in Excel VBA.
Why Change Column Widths in Excel VBA?
Before we dive into the nitty-gritty, let's quickly discuss why changing column widths in Excel VBA is important. Adjusting column widths can:
- Improve readability: Properly sized columns can make your data easier to read and understand.
- Enhance visualization: Well-sized columns can help highlight important data and trends.
- Increase efficiency: By adjusting column widths, you can reduce the need for horizontal scrolling and make it easier to navigate your spreadsheet.
Method 1: Using the ColumnWidth Property
One of the most straightforward ways to change column widths in Excel VBA is by using the ColumnWidth property. This property allows you to set the width of a column in points.
Sub ChangeColumnWidth()
' Change the width of column A to 10 points
Columns("A").ColumnWidth = 10
End Sub
In this example, we're changing the width of column A to 10 points. You can replace "A" with any column letter or number to adjust the width of a different column.
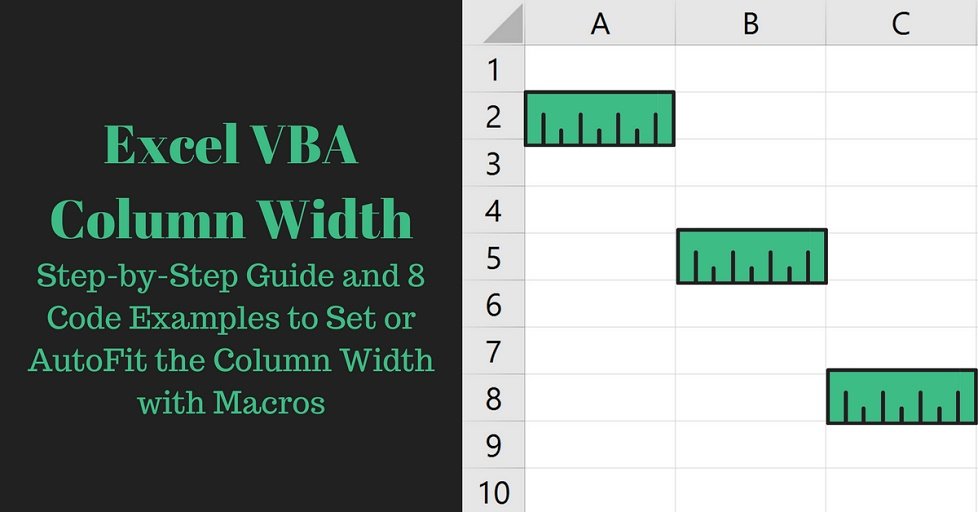
Method 2: Using the AutoFit Method
Another way to change column widths in Excel VBA is by using the AutoFit method. This method automatically adjusts the width of a column to fit the contents of the cells.
Sub AutoFitColumns()
' AutoFit the width of all columns in the active worksheet
ActiveSheet.Cells.AutoFit
End Sub
In this example, we're using the AutoFit method to adjust the width of all columns in the active worksheet. You can also specify a specific range of cells to AutoFit by replacing ActiveSheet.Cells
with Range("A1:C10")
, for example.
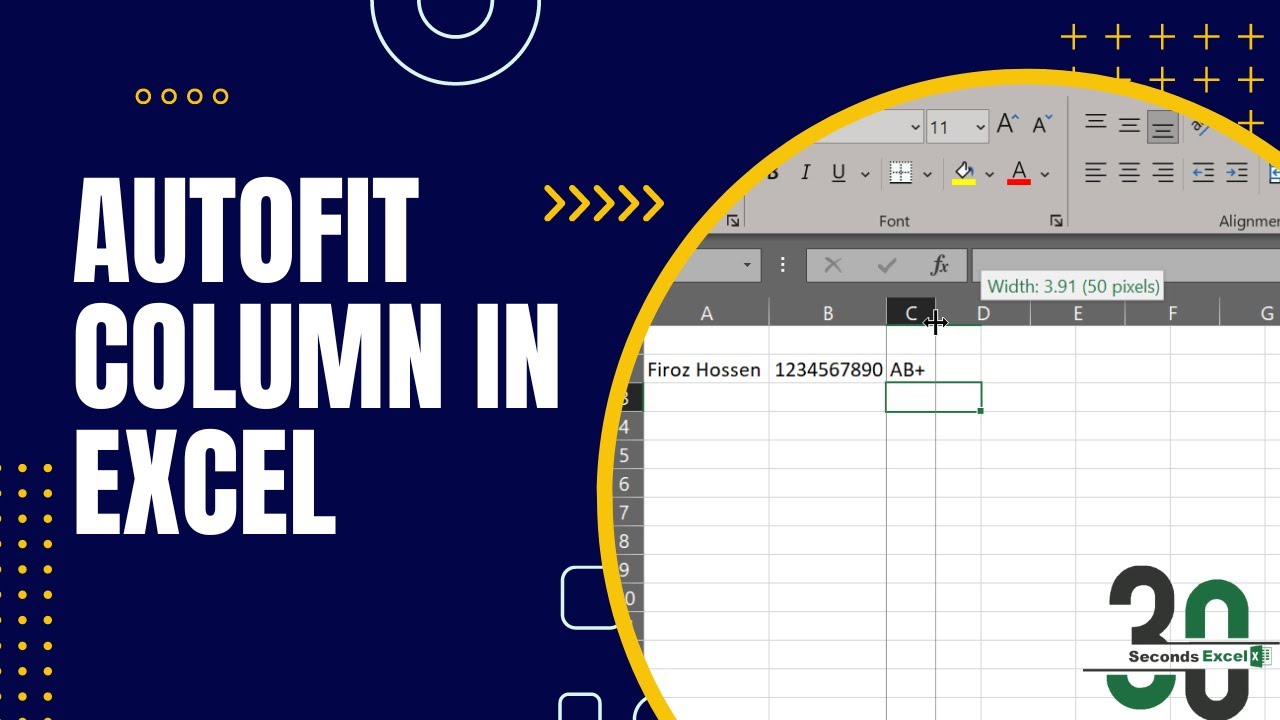
Method 3: Using the AutoFit Method with a Specific Range
As mentioned earlier, you can use the AutoFit method to adjust the width of a specific range of columns.
Sub AutoFitColumnsInRange()
' AutoFit the width of columns A to C
Range("A:C").AutoFit
End Sub
In this example, we're using the AutoFit method to adjust the width of columns A to C.
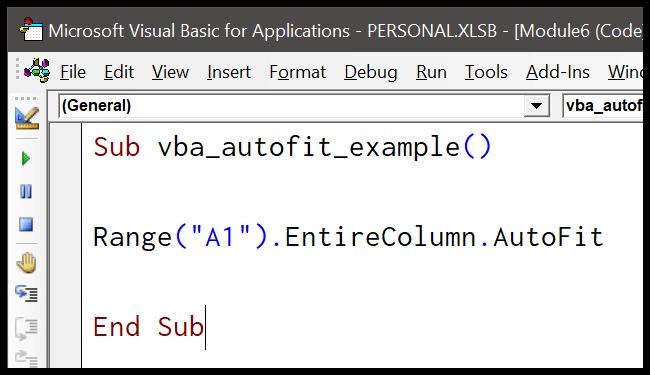
Method 4: Using a Loop to Adjust Multiple Columns
If you need to adjust the width of multiple columns, you can use a loop to iterate through each column and set the width.
Sub AdjustMultipleColumns()
' Adjust the width of columns A, C, and E
Dim i As Integer
For i = 1 To 3
Select Case i
Case 1
Columns("A").ColumnWidth = 10
Case 2
Columns("C").ColumnWidth = 15
Case 3
Columns("E").ColumnWidth = 20
End Select
Next i
End Sub
In this example, we're using a loop to adjust the width of columns A, C, and E.
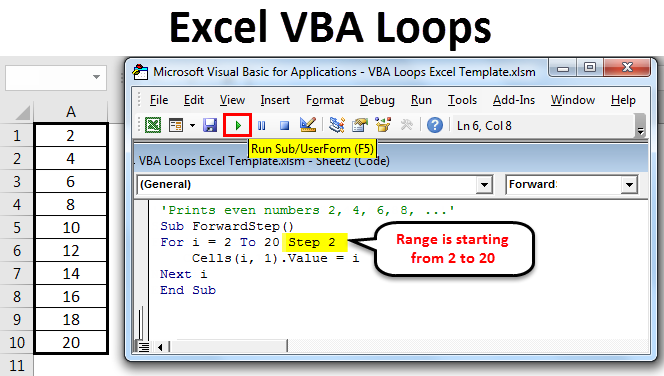
Method 5: Using a User-Defined Function
Finally, you can create a user-defined function to adjust the width of a column.
Function SetColumnWidth(col As String, width As Double) As Boolean
' Set the width of a column
Columns(col).ColumnWidth = width
SetColumnWidth = True
End Function
In this example, we're creating a user-defined function called SetColumnWidth
that takes two arguments: the column letter and the desired width. You can call this function from another procedure to adjust the width of a column.
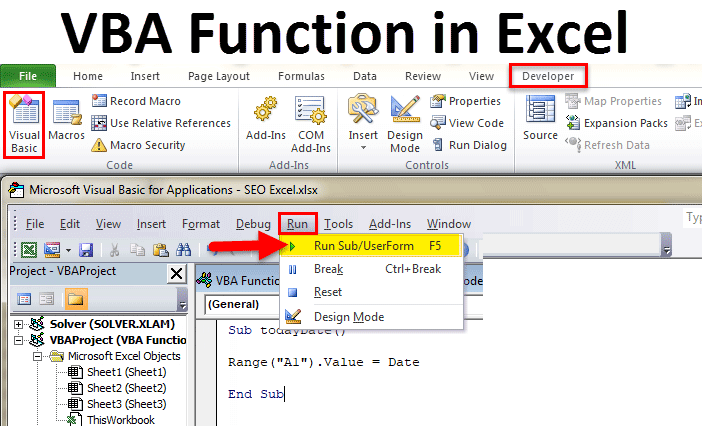
Gallery of Excel VBA Column Width Examples
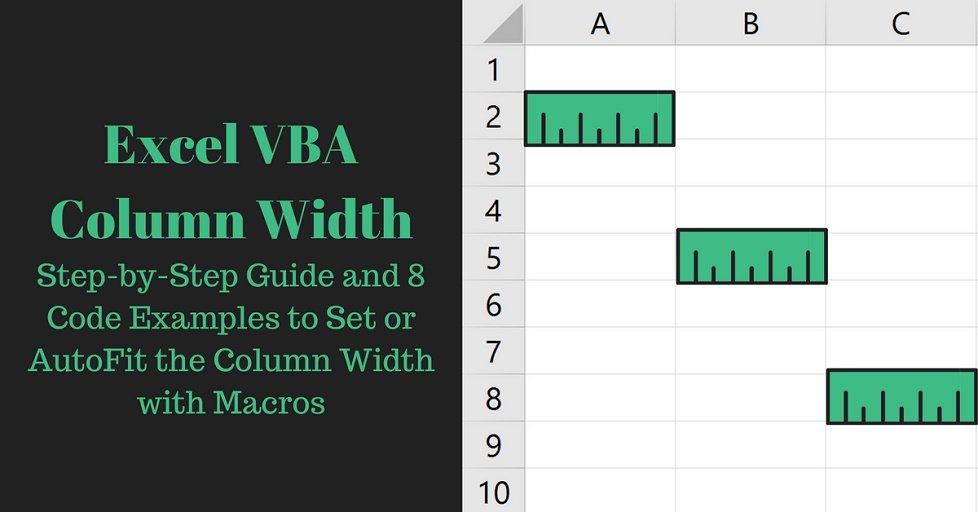
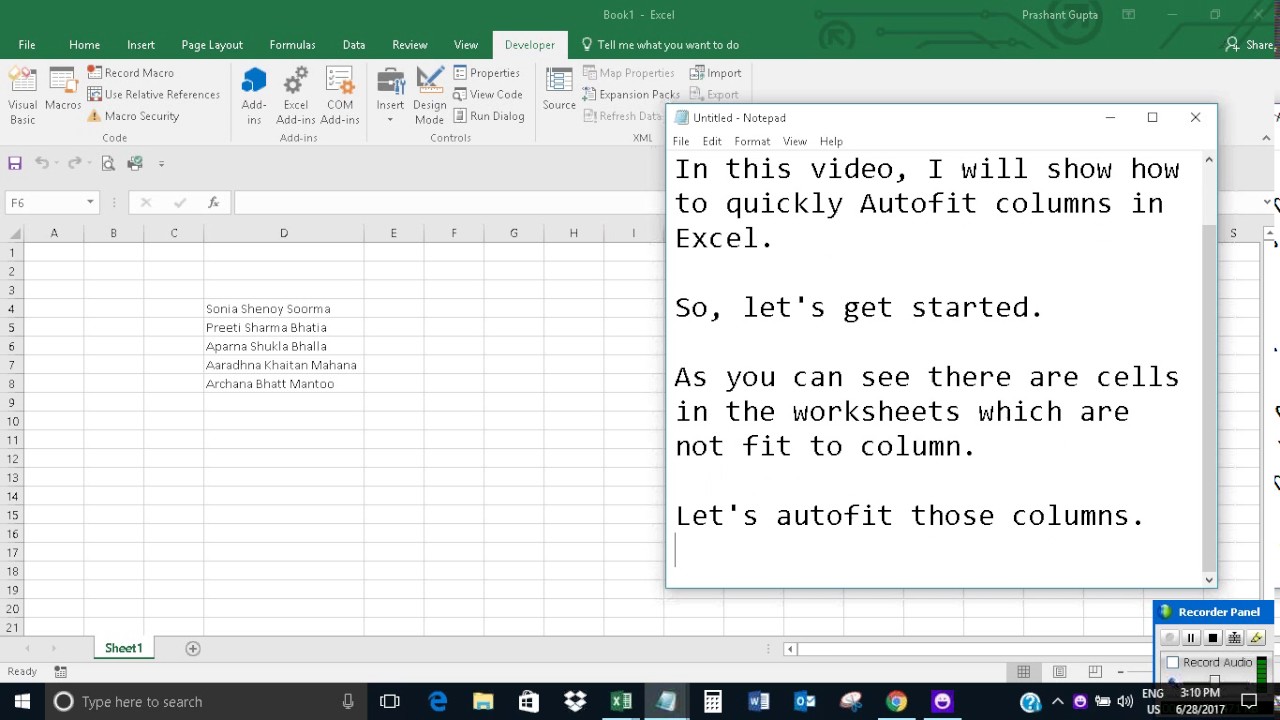
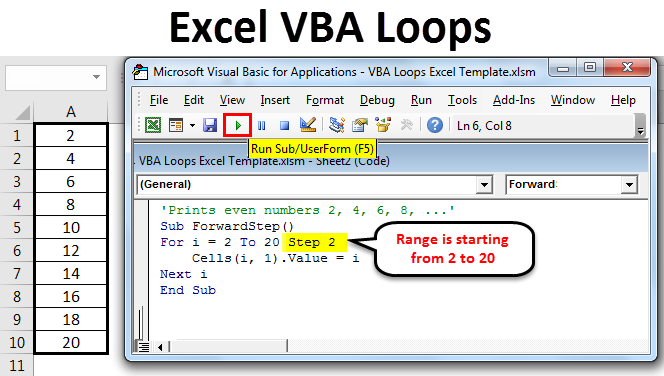
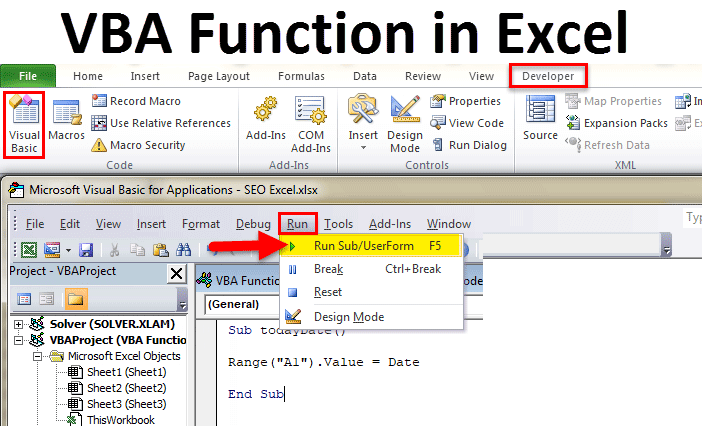
FAQs
How do I adjust the width of a column in Excel VBA?
+You can adjust the width of a column in Excel VBA by using the ColumnWidth property or the AutoFit method.
Can I use a loop to adjust the width of multiple columns?
+Yes, you can use a loop to iterate through each column and set the width.
How do I create a user-defined function to adjust the width of a column?
+You can create a user-defined function by defining a function with two arguments: the column letter and the desired width.
By following these five methods, you can easily change column widths in Excel VBA and improve the overall appearance and functionality of your spreadsheet. Remember to use the ColumnWidth property, AutoFit method, loops, and user-defined functions to adjust column widths with ease.